Sometime in your life you might want your script to be able to accept command line argument as input. This post will teach you the most basic usage of Argparse library.
I only touch surface of this library if you would like to know more detail usage read docs.python.org for further instructions.
Installation is quite straightforward, use the command below to install the package. Refer to pypi.org for more installation details/help.
pip install argparse
You can test the installation simply run below command in python interactive mode. If you did not see any output after the command then your installation is successful.
import argparse

Basic Syntax
from argparse import ArgumentParser
# positional argument
parser = ArgumentParser()
parser.add_argument("<VariableName>", help="<Help Message display to user>")
# optional argument
parser = ArgumentParser()
parser.add_argument("--<VariableName>", help="<Help Message display to user>")
There are two kinds of argument. One is positional argument, the other is option argument. I will introduce positional argument first followed by option argument.
Positional argument
Positional argument is mandatory and it’s position could not be change. Use code below as example, you supply name as the first argument and age as second argument.
#! /usr/bin/python3
from argparse import ArgumentParser
parser = ArgumentParser()
parser.add_argument("name", help="What's your name?")
parser.add_argument("age", help="How old are you?")
args = parser.parse_args()
print(f"Hello {args.name}, and you are {args.age} years old.")

If you switch position, the program become weird. It still execute but the behavior is not what we expected.
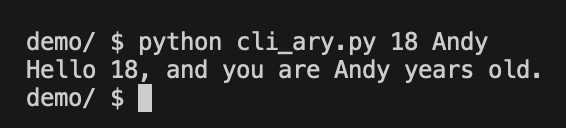
Optional argument
Optional argument is by its name optional, the value of the argument is depend on the flag before the value. Use below code as example, both age and name are optional argument.
Name could be specify with flag “–name” and age could be specify with flag “–age”. The position of arguments are not important.
(For demo purpose, I assume the user will provide no argument or 2 arguments.)
#! /usr/bin/python3
from argparse import ArgumentParser
parser = ArgumentParser()
parser.add_argument("--name", help="What's your name?")
parser.add_argument("--age", help="How old are you?")
args = parser.parse_args()
if args.name == None and args.age == None:
print(f"Hello Anonymous.")
else:
print(f"Hello {args.name}, and you are {args.age} year(s) old.")
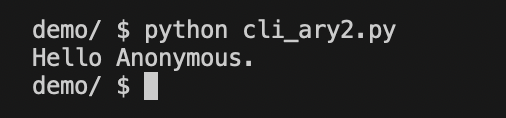


Help message
You can display help message to user and let them know what argument are expected. To show help message simply use “-h” flag.
python cli_ary.py -h
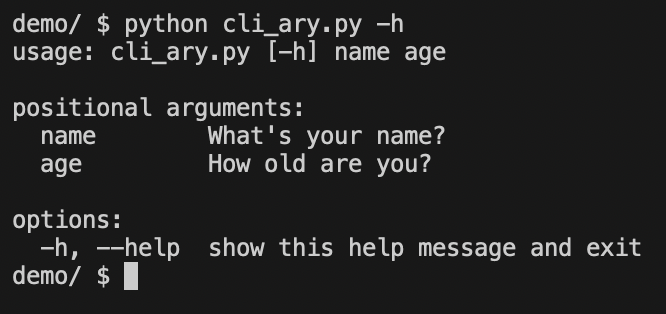
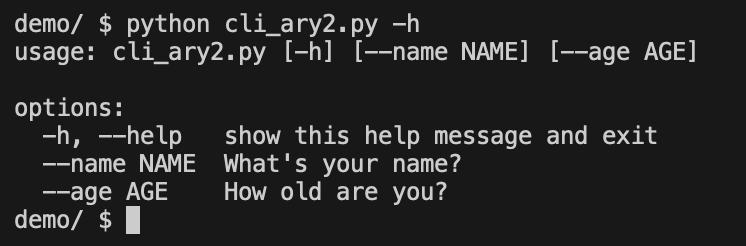